Dev Log - Multiplayer Social-Deduction Game #5
fifth and last in a series of dev logs for my connected games module.
🎮 Introduction
In this fifth update we are working on polishing the game. We have added multiple minigames to the clue collection as well as special abilites. For those of you just joining, you can catch up on our initial steps and challenges in Dev Log #1, Dev Log#2, Dev Log#3. Let’s get into the details.
🤝 NetworkEvents
One of the essential features of PUN2 is the RaiseEvent function. The base function looks as follows:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
using ExitGames.Client.Photon;
using Photon.Realtime;
using Photon.Pun;
public class SendEventExample
{
public const byte SomeEventCode = 1;
private void SendSomeEvent()
{
object[] content = new object[] { true, 2};
RaiseEventOptions raiseEventOptions =
new RaiseEventOptions { Receivers = ReceiverGroup.All };
PhotonNetwork.RaiseEvent(MoveUnitsToTargetPositionEventCode,
content, raiseEventOptions, SendOptions.SendReliable);
}
}
The function’s first parameter is the byte corresponding to the event to raise, the second is an object or object array for the content of the event. The subscribers then use the IOnEventCallback to receive these events.
1
2
3
4
5
6
7
8
9
public void OnEvent(EventData photonEvent)
{
byte eventCode = photonEvent.Code;
if (eventCode == SomeEventCode)
{
object[] data = (object[])photonEvent.CustomData;
bool someConditionIsTrue = (bool)data[0];
//rest of the implementation...
I wanted to refactor this approach, mainly to address the safety of classes raising these events and casting the content object. To do this I encapsulated this functionality into a single class with static delegates.

Firstly, the class inherits from my Singleton class. This is a base MonoBehaviour that implements the singleton initialisation. This ensures only one NetworkEvents object exists. Secondly, we implement IOnEventCallbacks to receive the callbacks. We also preemptively create RaiseEventOptions variables that we will re-use.
Next, we need to declare all of our event codes as follows:
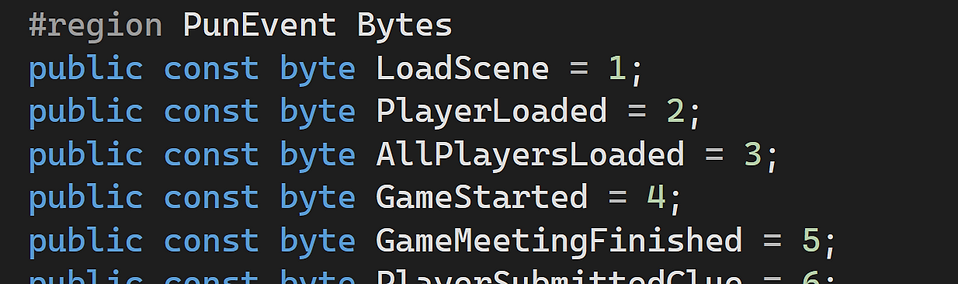
Rather than having classes directly call the Photon RaiseEvent function, we add static functions to this class for the objects to call. With the function parameters, we ensure that the event is always raised with correctly formatted data
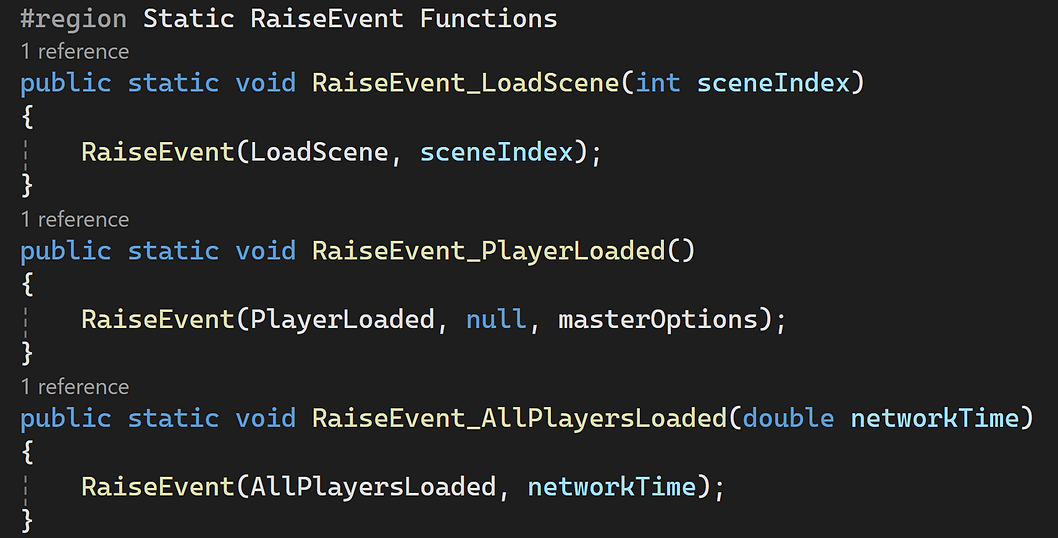
For the subscribers, we create a corresponding custom delegate:

From the interface Callback we can now invoke this delegate with reassurance that our content object is formatted correctly
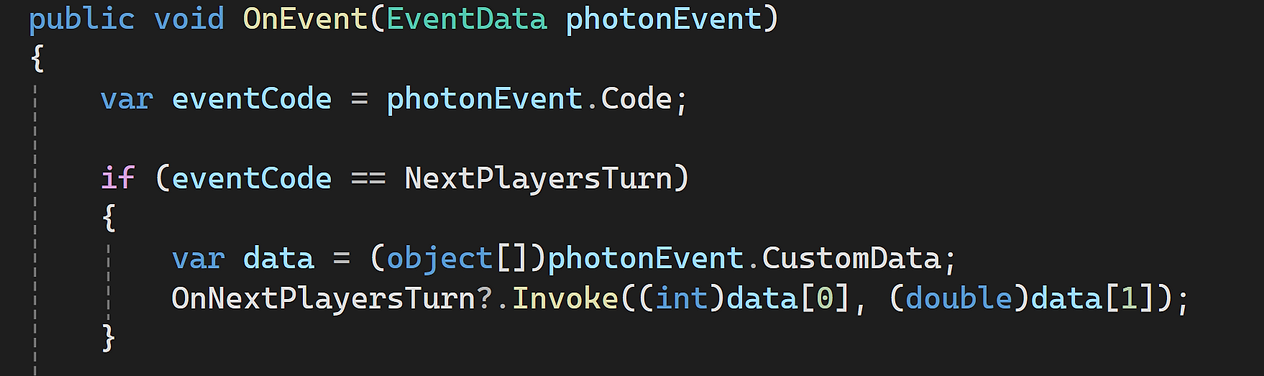
This is repeated for all of our custom events. Other objects now simply subscribe to the static events. Since the NetworkEvents class is raising and receiving the Photon events the datacasting is handled internally. This means there is less refactoring to do if we change an event and ensures other objects are not potentially trying to cast the data incorrectly.
🖥️ MiniGames
In addition to refactoring the networking code, I’ve added in MiniGames for the clue collection mechanic of the game. The games themselves are not networked and so the approach we use is to load a separate scene additively:
Each clue has an ‘AnalyseTool’ which from a gameplay perspective corresponds to a minigame. When interacting with a clue we call the following function:
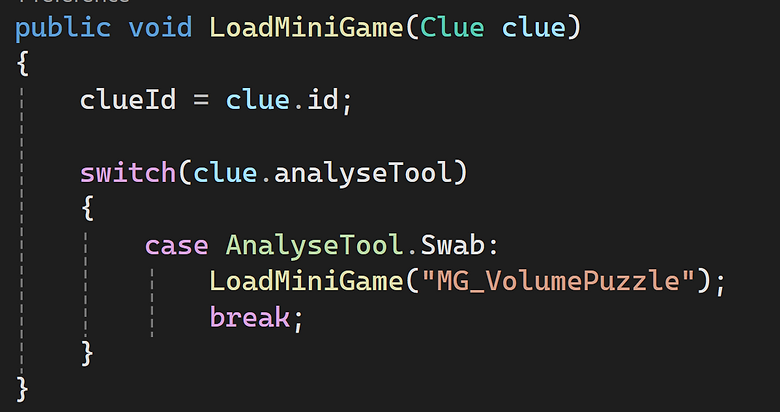
The LoadMiniGame function then checks for the scene and loads it in additively. I am also accessing my InputManager and switching the ActionMap to UI. This effectively stops input from being sent to the pawn in the game scene:
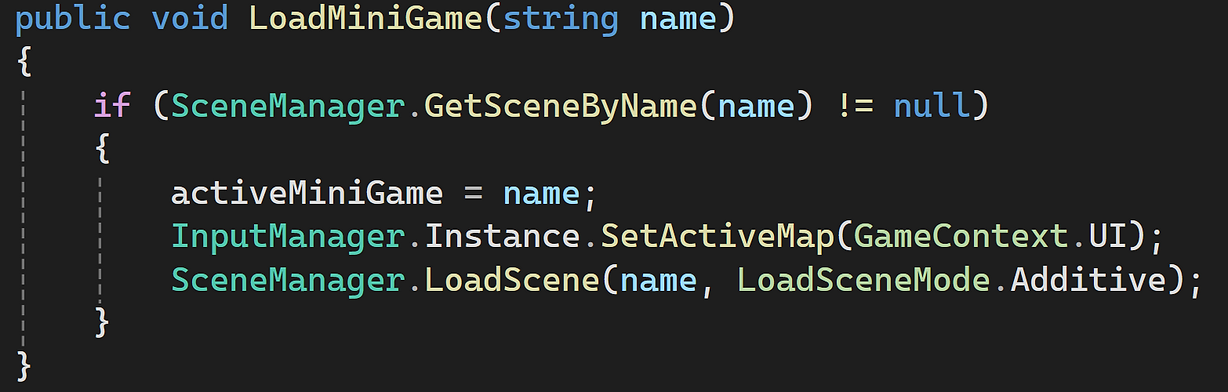
The final step is to unload the MiniGame when it is finished. For this, we use a separate function that takes the status of the game as a parameter we can then invoke the event and let the scoring system and inventory know if the player has successfully completed the MiniGame:
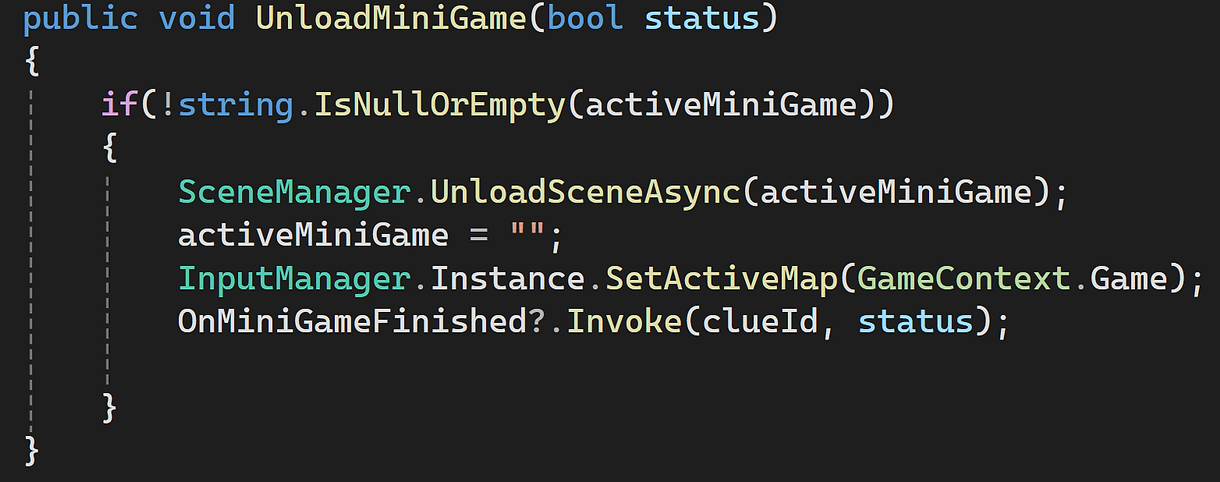
⚙️ Character Profile Menu
I also started work on a customisation menu. The basic setup lets the player cycle between the available character models and profile icons.
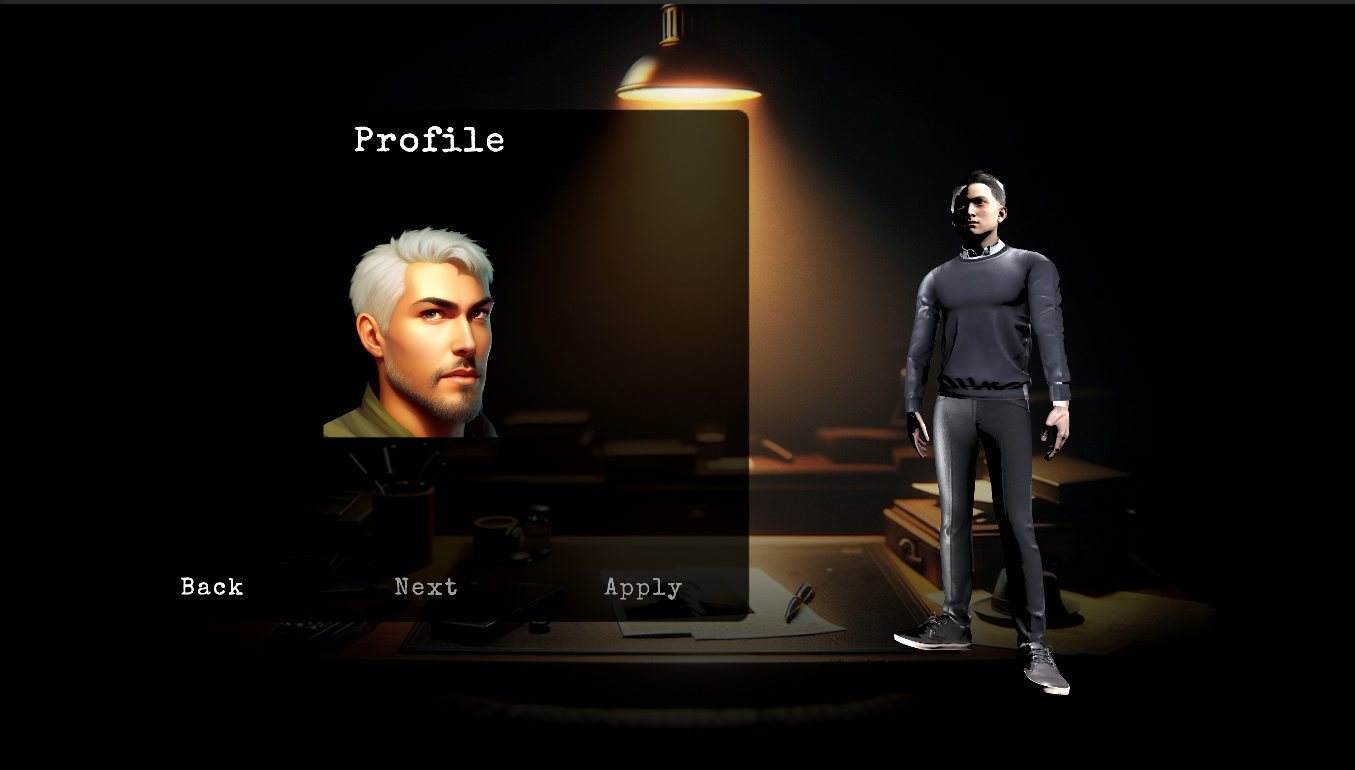
To save these values the models and icons are indexed and saved to a UserProfile object. This data is loaded when we open the profile menu

When changes are made the player can hit the apply button to call the following function:

The SaveData class is a custom class that converts objects to JSON and saves them with the System.IO namespace. Once the profile is saved we Invoke the OnUserProfileUpdated event which in turn updates the photon player.
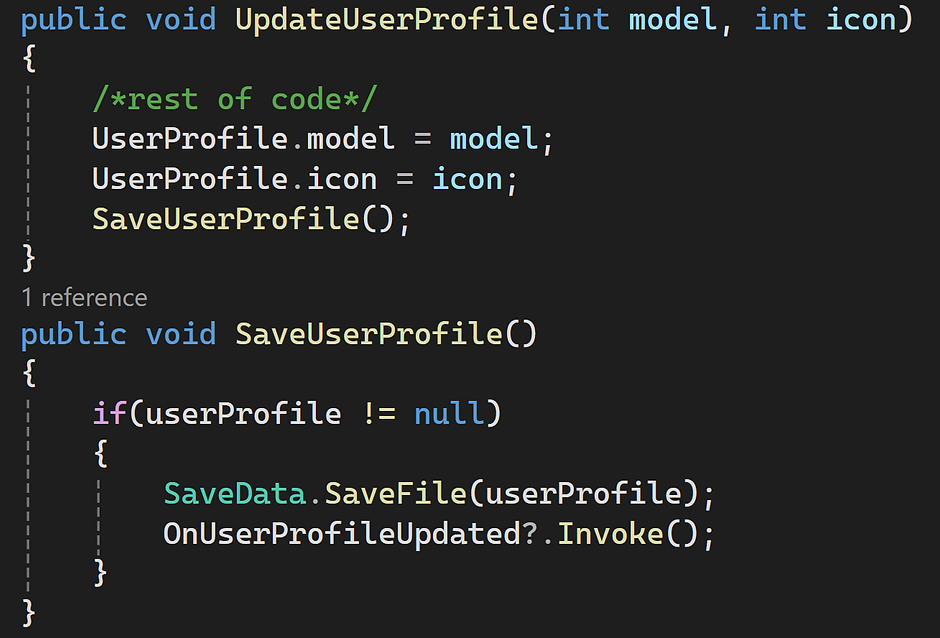
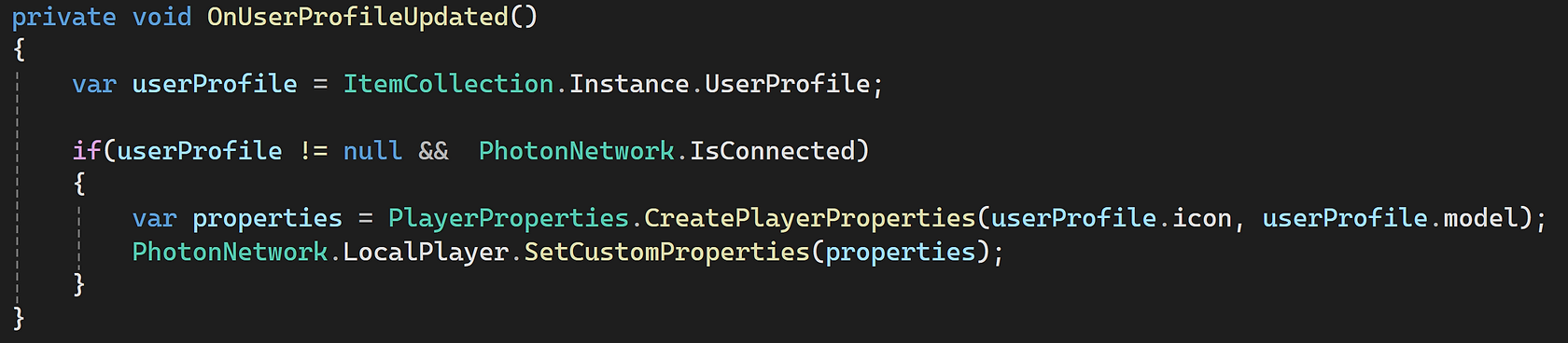
From this callback, we set the values to the CustomProperties of the Photon Player. Once the player joins a room these values can then be read by other clients and displayed for the player.

📌 Next Steps
With most of the larger systems in place the next steps will be refining the UI as well adding minigames for the clue collecting and abilities #